If you’re using any of the out-of-the-box mvc template projects (Visual Studio 2012 / c#), they do a great job with including functionality for user membership including log in, register and change password. However it does seem to be missing a ‘forgot password’ or ‘reset password’ function. Below is how you can quickly add this functionality by modifying the existing controllers and views:
1. Add a new view (I’m calling mine ResetPass) under the Views > Account folder:
<%@ Page Title="" Language="C#" MasterPageFile="~/Views/Shared/Site.Master" Inherits="System.Web.Mvc.ViewPage<ledme2.Models.LoginModel>" %>
<asp:Content ID="Content1" ContentPlaceHolderID="TitleContent" runat="server">
Reset Pass
</asp:Content>
<asp:Content ID="Content2" ContentPlaceHolderID="MainContent" runat="server">
<hgroup class="title">
<h1>Reset Your Password</h1>
</hgroup>
<section id="loginForm">
<h2>Enter the username you wish to reset. An email will be sent with your password and the new password will expire in 3 hours.</h2>
<% using (Html.BeginForm(new { ReturnUrl = ViewBag.ReturnUrl }))
{ %>
<%: Html.AntiForgeryToken() %>
<%: Html.ValidationSummary(true) %>
<fieldset>
<legend>Enter the username you wish to reset. An email will be sent with your password and the new password will expire in 3 hours.</legend>
<ol>
<li>
<%: Html.LabelFor(m => m.UserName) %>
<%: Html.TextBoxFor(m => m.UserName) %>
<%: Html.ValidationMessageFor(m => m.UserName) %>
</li>
</ol>
<input type="submit" value="Reset" />
</fieldset>
<% } %>
<% if (ViewData["msg"] != null)
{ %>
<%: ViewData["msg"] %>
<% } %>
</section>
</asp:Content>
<asp:Content ID="Content3" ContentPlaceHolderID="FeaturedContent" runat="server">
</asp:Content>
<asp:Content ID="Content4" ContentPlaceHolderID="ScriptsSection" runat="server">
</asp:Content>
2. Add these two methods to the AccountController.cs (I’ve left out the code w/ the email function, feel free to add your own):
[AllowAnonymous]
public ActionResult ResetPass(string returnUrl)
{
ViewBag.ReturnUrl = returnUrl;
return View();
}
[HttpPost]
[AllowAnonymous]
[ValidateAntiForgeryToken]
public ActionResult ResetPass(LoginModel model, string returnUrl)
{
if (WebSecurity.UserExists(model.UserName))
{
string ptoken = WebSecurity.GeneratePasswordResetToken(model.UserName, 190);
//change to be as secure as you choose
string tmpPass = Membership.GeneratePassword(10, 4);
WebSecurity.ResetPassword(ptoken, tmpPass);
//add your own email logic here
ViewData["msg"] = "Your new password is: " + tmpPass;
return View(model);
}
else
{
ModelState.AddModelError("", "The user name wasn't found.");
}
return View(model);
}
3. Add this code to the Login.aspx view:
<p>
<%: Html.ActionLink("Reset", "ResetPass") %> your forgotten passord?
</p>
4. Now you should get this result on the login page:
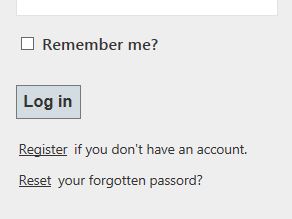
And when clicked:
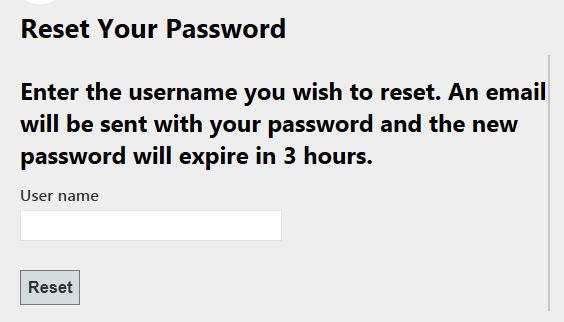